Cavities and Resonators¶
Ring Resonators¶
-
class
picwriter.components.
Ring
(wgt, radius, coupling_gap, wrap_angle=0, parity=1, draw_bus_wg=True, port=(0, 0), direction=u'EAST')¶ Ring Resonator Cell class.
- Args:
- wgt (WaveguideTemplate): WaveguideTemplate object
- radius (float): Radius of the resonator
- coupling_gap (float): Distance between the bus waveguide and resonator
- Keyword Args:
- wrap_angle (float): Angle in radians between 0 and pi that determines how much the bus waveguide wraps along the resonator. 0 corresponds to a straight bus waveguide, and pi corresponds to a bus waveguide wrapped around half of the resonator. Defaults to 0.
- parity (1 or -1): If 1, resonator to left of bus waveguide, if -1 resonator to the right
- port (tuple): Cartesian coordinate of the input port (x1, y1)
- direction (string): Direction that the component will point towards, can be of type ‘NORTH’, ‘WEST’, ‘SOUTH’, ‘EAST’, OR an angle (float, in radians)
- draw_bus_wg (bool): If False, does not generate the bus waveguide. Instead, the input/output port positions will be at the some location at the bottom of the ring, and the user can route their own bus waveguide. Defaults to True.
- Members:
- portlist (dict): Dictionary with the relevant port information
- Portlist format:
- portlist[‘input’] = {‘port’: (x1,y1), ‘direction’: ‘dir1’}
- portlist[‘output’] = {‘port’: (x2, y2), ‘direction’: ‘dir2’}
Where in the above (x1,y1) is the same as the ‘port’ input, (x2, y2) is the end of the component, and ‘dir1’, ‘dir2’ are of type ‘NORTH’, ‘WEST’, ‘SOUTH’, ‘EAST’, or an angle in radians. ‘Direction’ points towards the waveguide that will connect to it.
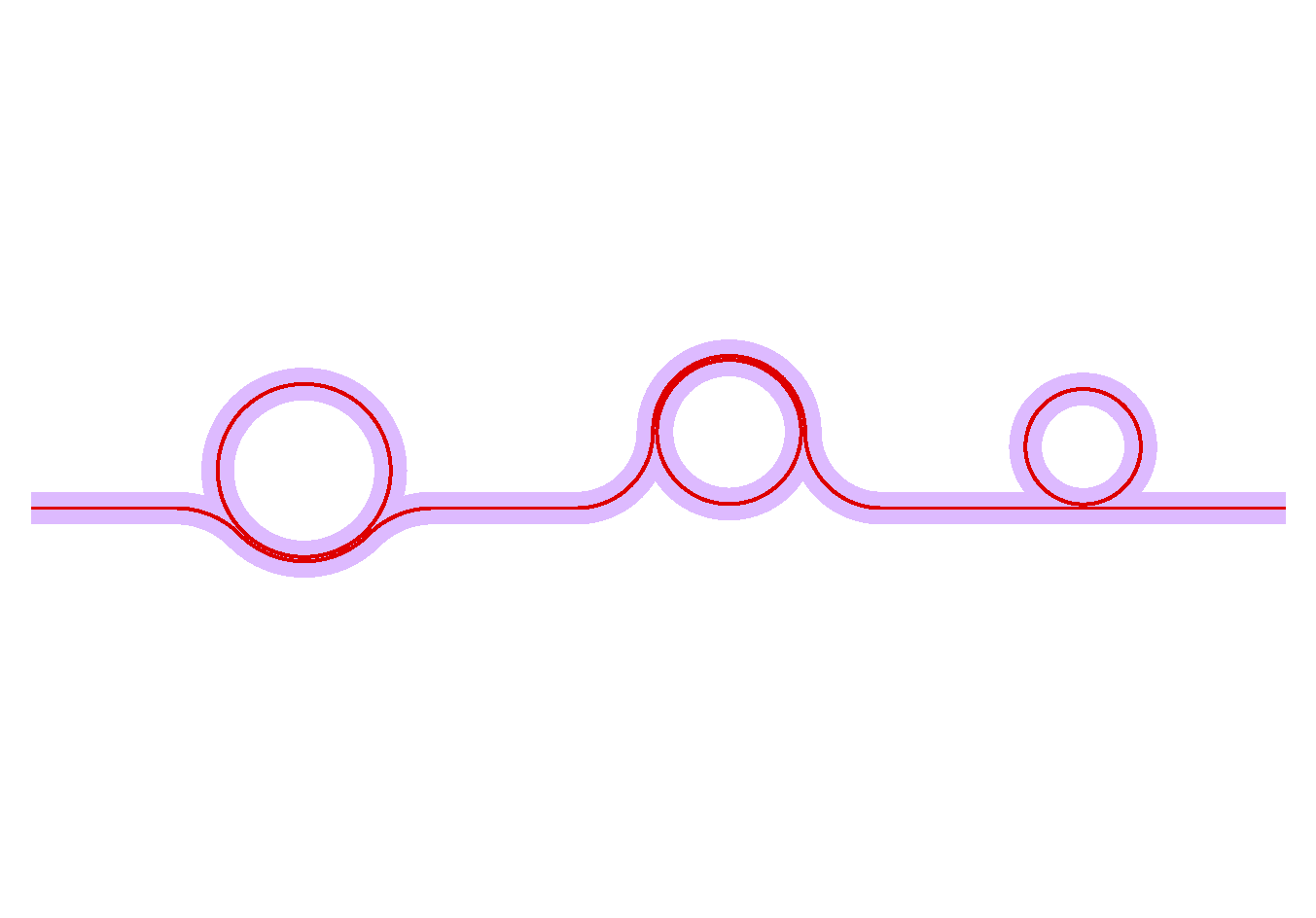
The code for generating the above three rings is:
top = gdspy.Cell("top")
wgt = WaveguideTemplate(bend_radius=50, resist='+')
wg1=Waveguide([(0,0), (100,0)], wgt)
tk.add(top, wg1)
r1 = Ring(wgt, 60.0, 1.0, wrap_angle=np.pi/2., parity=1, **wg1.portlist["output"])
wg2=Waveguide([r1.portlist["output"]["port"], (r1.portlist["output"]["port"][0]+100, r1.portlist["output"]["port"][1])], wgt)
tk.add(top, wg2)
r2 = Ring(wgt, 50.0, 0.8, wrap_angle=np.pi, parity=-1, **wg2.portlist["output"])
wg3=Waveguide([r2.portlist["output"]["port"], (r2.portlist["output"]["port"][0]+100, r2.portlist["output"]["port"][1])], wgt)
tk.add(top, wg3)
r3 = Ring(wgt, 40.0, 0.6, parity=1, **wg3.portlist["output"])
wg4=Waveguide([r3.portlist["output"]["port"], (r3.portlist["output"]["port"][0]+100, r3.portlist["output"]["port"][1])], wgt)
tk.add(top, wg4)
tk.add(top, r1)
tk.add(top, r2)
tk.add(top, r3)
gdspy.LayoutViewer()
Disk Resonators¶
-
class
picwriter.components.
Disk
(wgt, radius, coupling_gap, wrap_angle=0, parity=1, port=(0, 0), direction=u'EAST')¶ Disk Resonator Cell class.
- Args:
- wgt (WaveguideTemplate): WaveguideTemplate object
- radius (float): Radius of the disk resonator
- coupling_gap (float): Distance between the bus waveguide and resonator
- Keyword Args:
- wrap_angle (float): Angle in radians between 0 and pi (defaults to 0) that determines how much the bus waveguide wraps along the resonator. 0 corresponds to a straight bus waveguide, and pi corresponds to a bus waveguide wrapped around half of the resonator.
- parity (1 or -1): If 1, resonator to left of bus waveguide, if -1 resonator to the right
- port (tuple): Cartesian coordinate of the input port (x1, y1)
- direction (string): Direction that the component will point towards, can be of type ‘NORTH’, ‘WEST’, ‘SOUTH’, ‘EAST’, OR an angle (float, in radians)
- Members:
- portlist (dict): Dictionary with the relevant port information
- Portlist format:
- portlist[‘input’] = {‘port’: (x1,y1), ‘direction’: ‘dir1’}
- portlist[‘output’] = {‘port’: (x2, y2), ‘direction’: ‘dir2’}
Where in the above (x1,y1) is the same as the ‘port’ input, (x2, y2) is the end of the component, and ‘dir1’, ‘dir2’ are of type ‘NORTH’, ‘WEST’, ‘SOUTH’, ‘EAST’, or an angle in radians. ‘Direction’ points towards the waveguide that will connect to it.
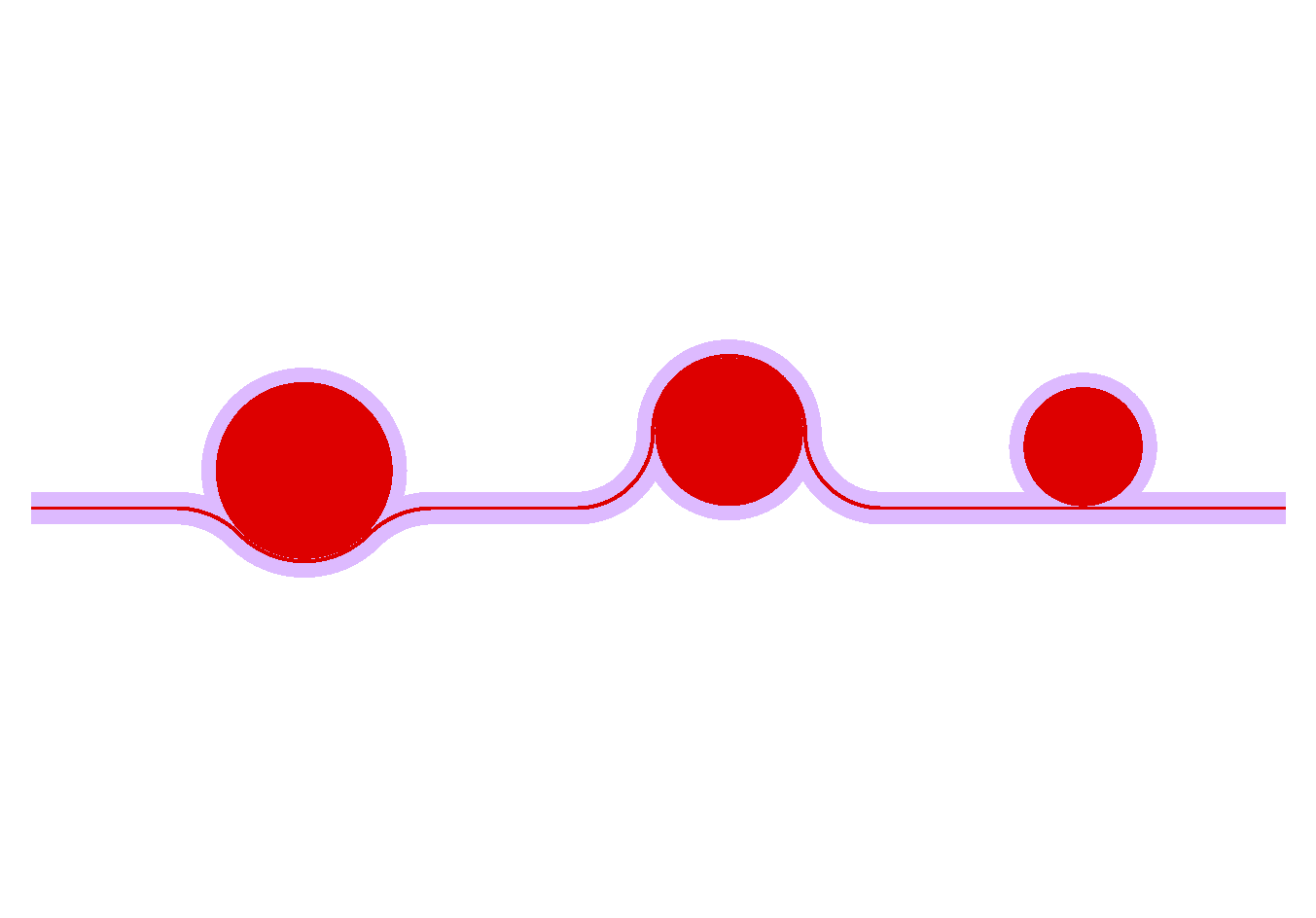
The code for generating the above three disks is:
top = gdspy.Cell("top")
wgt = WaveguideTemplate(bend_radius=50, resist='+')
wg1=Waveguide([(0,0), (100,0)], wgt)
tk.add(top, wg1)
r1 = Disk(wgt, 60.0, 1.0, wrap_angle=np.pi/2., parity=1, **wg1.portlist["output"])
wg2=Waveguide([r1.portlist["output"]["port"], (r1.portlist["output"]["port"][0]+100, r1.portlist["output"]["port"][1])], wgt)
tk.add(top, wg2)
r2 = Disk(wgt, 50.0, 0.8, wrap_angle=np.pi, parity=-1, **wg2.portlist["output"])
wg3=Waveguide([r2.portlist["output"]["port"], (r2.portlist["output"]["port"][0]+100, r2.portlist["output"]["port"][1])], wgt)
tk.add(top, wg3)
r3 = Disk(wgt, 40.0, 0.6, parity=1, **wg3.portlist["output"])
wg4=Waveguide([r3.portlist["output"]["port"], (r3.portlist["output"]["port"][0]+100, r3.portlist["output"]["port"][1])], wgt)
tk.add(top, wg4)
tk.add(top, r1)
tk.add(top, r2)
tk.add(top, r3)
gdspy.LayoutViewer()